How do you manage state in an Angular application?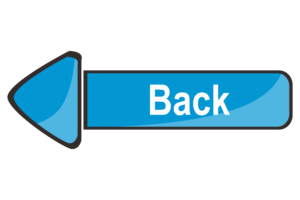
Chapter 1: Understanding State Management in Angular Applications
Overview of State Management
State management is a crucial aspect of developing robust and efficient Angular applications. It involves managing the state of your application, which includes data that needs to be shared across different components, modules, or services. In this subchapter, we will provide an overview of state management in Angular and discuss best practices, tools, and strategies to effectively manage state in your applications.
One of the most popular tools for state management in Angular is NgRx, which is inspired by Redux. NgRx provides a set of libraries that help you manage the state of your application in a predictable and efficient way. By using NgRx, you can centralize your application’s state, make state changes predictable, and improve the performance of your application.
Implementing Redux in Angular for state management involves defining actions, reducers, and selectors to manage the state of your application. Redux helps you maintain a single source of truth for your application’s state and enables you to easily debug and test your application.
Managing authentication state in Angular applications is another important aspect of state management. You can use services or NgRx to manage the authentication state of your application. By centralizing the authentication state, you can easily check the user’s authentication status throughout your application.
State management with RxJS in Angular is another popular approach to managing the state of your application. RxJS provides a set of tools and operators that help you handle complex state transitions, manage asynchronous data streams, and optimize the performance of your application.
In this subchapter, we will also discuss the best practices for state management in Angular, including using services vs. NgRx, optimizing performance with efficient state management, managing global vs. local state, and state management patterns for Angular components. By following these best practices and using the right tools and strategies, you can effectively manage the state of your Angular applications and build scalable and maintainable software.Importance of State Management in Angular
State management is a crucial aspect of developing robust and efficient Angular applications. It plays a vital role in ensuring that the application’s data is handled in a consistent and organized manner, making it easier to debug and maintain the codebase. In this subchapter, we will delve into the importance of state management in Angular and how it can benefit Angular programmers.
One of the key reasons why state management is essential in Angular is that it helps in maintaining a single source of truth for the application’s data. By centralizing the management of the application’s state, developers can avoid inconsistencies and conflicts that often arise when multiple components try to update the same data simultaneously. This leads to a more predictable and stable application behavior, making it easier to track down and fix bugs.
Furthermore, effective state management in Angular applications can improve the overall performance of the application. By carefully managing the data flow and minimizing unnecessary re-renders, developers can ensure that the application runs smoothly and efficiently, even when handling large amounts of data.
State management also plays a crucial role in managing authentication state in Angular applications. By keeping track of the user’s authentication status and permissions, developers can control access to different parts of the application and provide a seamless and secure user experience.
In this subchapter, we will explore various state management strategies and best practices for Angular programmers, including using NgRx and implementing Redux in Angular applications. We will also discuss how to handle complex state transitions, optimize performance, and manage global vs. local state effectively in Angular applications. By mastering these state management patterns and techniques, Angular programmers can build scalable and maintainable applications that deliver a seamless user experience.Challenges in State Management
State management in Angular applications can be a complex and challenging task for developers. There are various challenges that developers may face when trying to effectively manage the state of their application. One of the biggest challenges is handling complex state transitions. As an Angular programmer, you may encounter situations where the state of your application needs to change in response to user interactions, server responses, or other external events. Managing these state transitions can be difficult, especially as your application grows in size and complexity.
Another challenge in state management is deciding between using services or NgRx for managing state. While services are a simple and straightforward way to manage state in Angular applications, NgRx provides a more robust and scalable solution. As an Angular programmer, you may need to weigh the benefits and drawbacks of each approach to determine which is best for your specific needs.
Managing global versus local state is another challenge that developers face when working with state management in Angular. Global state is shared across the entire application, while local state is specific to a particular component or module. Deciding where to store state and how to manage it effectively can be a challenging task.
In addition to these challenges, Angular programmers must also consider optimizing performance with efficient state management. Poorly managed state can lead to performance issues, such as slow rendering times and unresponsive user interfaces. By implementing best practices for state management and using tools like NgRx and RxJS, developers can optimize performance and ensure a smooth user experience.
Overall, state management in Angular applications presents a number of challenges for developers. By understanding these challenges and implementing best practices, Angular programmers can effectively manage state in their applications and create high-performing, responsive user interfaces.
Chapter 2: Best Practices for State Management in Angular Applications
Single Source of Truth
In the world of Angular development, managing state in your application is crucial for ensuring a smooth and efficient user experience. One key concept that all Angular programmers should be familiar with is the idea of a “Single Source of Truth.”
The Single Source of Truth principle states that there should be one definitive source of state within your application. This means that all components should rely on this single source for accessing and updating the state, rather than each component managing its own separate state. By centralizing your state management in this way, you can avoid inconsistencies and conflicts that can arise when different parts of your application have different ideas of what the current state should be.
To implement the Single Source of Truth principle in your Angular application, one popular approach is to use NgRx, a state management library inspired by Redux. NgRx provides a set of tools and patterns for managing state in a predictable and efficient way, allowing you to easily define and update your application’s state in a clear and organized manner.
By implementing NgRx in your Angular application, you can take advantage of features such as actions, reducers, and selectors to create a structured and maintainable state management system. This can help you handle complex state transitions, optimize performance, and manage both global and local state effectively.
While NgRx is a powerful tool for state management in Angular, it’s important to consider whether it is the best solution for your specific project. Depending on the size and complexity of your application, using services or other state management patterns may be more appropriate. Ultimately, the goal is to find the right balance between simplicity and scalability in managing state in your Angular application.Immutability
Immutability is a crucial concept in state management for Angular applications. In simple terms, immutability means that once an object is created, it cannot be changed. Instead, any modifications to the object result in a new object being created. This may seem like an unnecessary extra step, but it has significant benefits in terms of predictability and performance.
By using immutability in your state management strategy, you can ensure that changes to your application’s state are always explicit and traceable. This can help prevent bugs and make it easier to reason about how your application is behaving. Additionally, immutability can also help with performance optimization, as it allows Angular to more easily detect when changes have been made to the state and optimize the rendering process accordingly.
One popular tool for implementing immutability in Angular applications is NgRx. NgRx is a state management library that is heavily inspired by Redux, a popular state management library for React applications. By using NgRx, you can easily implement a unidirectional data flow in your Angular application, ensuring that changes to the state are always explicit and predictable.
When managing authentication state in Angular applications, immutability can be particularly useful. By using immutability to ensure that changes to the authentication state are always explicit and traceable, you can help prevent security vulnerabilities and make it easier to maintain and debug your application.
In conclusion, immutability is a powerful tool for state management in Angular applications. By using immutability to ensure that changes to your application’s state are always explicit and traceable, you can make your application more predictable, performant, and maintainable.Consistent State Updates
One of the key challenges in state management for Angular applications is ensuring that state updates are handled consistently throughout the application. In order to maintain a reliable and predictable state, it is important to establish best practices for managing state updates.
When working with state management in Angular, it is essential to follow a structured approach to state updates to avoid potential issues such as race conditions or inconsistent data. By implementing a consistent approach to state updates, developers can ensure that the application remains stable and performs optimally.
One popular strategy for achieving consistent state updates in Angular applications is to use NgRx, a reactive state management library inspired by Redux. NgRx provides a set of tools and patterns for managing state in a predictable and efficient manner, making it easier to handle complex state transitions and ensure that updates are handled consistently throughout the application.
Another approach to achieving consistent state updates in Angular is to implement Redux, a predictable state container that helps manage the state of an application in a centralized and predictable way. By following the principles of Redux, developers can ensure that state updates are handled consistently and that the application remains stable and performant.
In addition to using NgRx and Redux, developers can also leverage RxJS for state management in Angular applications. RxJS provides a powerful set of tools for handling asynchronous data streams, making it easier to manage state updates in a reactive and efficient manner.
Overall, maintaining consistent state updates is essential for ensuring the stability and performance of an Angular application. By following best practices for state management and leveraging tools such as NgRx, Redux, and RxJS, developers can achieve reliable and predictable state updates that enhance the overall user experience.
Chapter 3: Using NgRx for State Management in Angular
Introduction to NgRx
Welcome to the world of NgRx, a powerful state management library for Angular applications. As an Angular programmer, you may already be familiar with the challenges of managing state in complex applications. NgRx provides a solution by implementing the Redux pattern in Angular, allowing you to centralize and manage your application state with ease.
In this subchapter, we will introduce you to NgRx and explain why it is a popular choice for state management in Angular applications. We will discuss best practices for state management in Angular, including the use of immutable data structures and the importance of separating concerns in your application.
You will learn how to use NgRx to implement the Redux pattern in your Angular application, allowing you to manage your application state in a predictable and efficient manner. We will walk you through the process of setting up NgRx in your project and show you how to create actions, reducers, and effects to handle state changes.
We will also cover topics such as managing authentication state in Angular applications, handling complex state transitions, and optimizing performance with efficient state management techniques. You will learn the differences between using services and NgRx for state management, as well as how to manage global versus local state in your Angular application.
By the end of this subchapter, you will have a solid understanding of how to use NgRx for state management in your Angular applications, allowing you to build scalable and maintainable applications with ease. Let’s dive in and explore the world of NgRx together!Setting up NgRx in Angular
NgRx is a powerful state management library for Angular applications, based on the principles of Redux. By using NgRx, you can centralize and manage the state of your Angular application in a predictable and efficient way. In this subchapter, we will guide you through the process of setting up NgRx in your Angular project.
To start using NgRx in your Angular application, you first need to install the necessary packages. You can do this by running the following command in your terminal:
“`npm install @ngrx/store @ngrx/effects @ngrx/entity @ngrx/router-store @ngrx/store-devtools“`
Once you have installed the required packages, you need to set up the NgRx store in your Angular application. This involves creating reducers, actions, effects, and selectors to manage the state of your application.
Reducers are pure functions that specify how the state of your application changes in response to actions. Actions are payloads of information that are sent to the store to update the state. Effects are used to handle side effects, such as making API calls or interacting with external services. Selectors are used to retrieve specific pieces of state from the store.
After setting up the NgRx store, you can start dispatching actions to update the state of your application. By following the principles of Redux and using NgRx, you can ensure that your Angular application’s state is managed in a consistent and efficient manner.
In the next chapters, we will delve deeper into advanced NgRx concepts and best practices for state management in Angular applications. Stay tuned for more insights on optimizing performance, managing global vs. local state, and handling complex state transitions in your Angular projects.Actions, Reducers, and Effects in NgRx
In the world of Angular development, managing state effectively is crucial for building robust and maintainable applications. This is where tools like NgRx come into play, offering a powerful solution for state management through actions, reducers, and effects.
Actions in NgRx are simple objects that represent unique events in your application. These actions are dispatched to trigger state changes, making it easy to track and manage the flow of data within your application. By defining actions for specific events, you can ensure that your application’s state remains consistent and predictable.
Reducers, on the other hand, are pure functions that take the current state and an action as input, and return a new state based on that action. By using reducers to handle state changes, you can keep your application’s data immutable and easily traceable. This helps to avoid bugs and make it easier to debug and test your code.
Effects in NgRx are used for handling side effects, such as asynchronous operations like fetching data from an API. By encapsulating these side effects within effects, you can keep your reducers pure and focused on state management. This separation of concerns makes your code more maintainable and easier to reason about.
In this subchapter, we will explore how to implement actions, reducers, and effects in NgRx to effectively manage state in your Angular applications. We will discuss best practices for using these tools, as well as tips for optimizing performance and handling complex state transitions. By the end of this subchapter, you will have a solid understanding of how to leverage NgRx for state management in your Angular projects, allowing you to build scalable and maintainable applications with ease.
Chapter 4: Implementing Redux in Angular for State Management
Redux Principles
In the world of Angular programming, understanding and implementing Redux principles is crucial for effective state management in applications. Redux is a predictable state container that helps manage the state of an application in a consistent and efficient manner. By following Redux principles, Angular developers can ensure that their applications are well-structured, maintainable, and performant.
One of the key principles of Redux is the concept of a single source of truth. This means that the state of an application is stored in a single object, known as the store, which is immutable. Any changes to the state must be done through pure functions called reducers, which take the current state and an action as input and return a new state. This ensures that state changes are predictable and traceable.
Another important principle of Redux is the idea of pure functions. Reducers in Redux are pure functions, meaning that they produce the same output for the same input and have no side effects. This makes it easier to test and debug state changes in an application.
When implementing Redux in an Angular application, developers can use NgRx, a library that provides tools and patterns for managing state using Redux principles. NgRx includes features such as actions, reducers, selectors, and effects, which help streamline the state management process.
By following best practices for state management in Angular applications, such as using NgRx for complex state transitions, handling authentication state, and optimizing performance, developers can ensure that their applications are well-architected and maintainable. By understanding and implementing Redux principles, Angular programmers can take their state management strategies to the next level and build robust and scalable applications.Integrating Redux with Angular
Integrating Redux with Angular can greatly enhance the state management capabilities of your application. Redux is a predictable state container for JavaScript apps that helps you manage the state of your application in a single, centralized location. By integrating Redux with Angular, you can create a more organized and efficient way to handle state changes in your application.
To integrate Redux with Angular, you can use the NgRx library, which provides a set of tools and techniques for managing state in Angular applications using Redux principles. NgRx allows you to define actions, reducers, and selectors to handle state changes in a predictable and consistent manner.
Implementing Redux in Angular for state management involves setting up the store, defining actions and reducers, and using selectors to access the state data in your components. By following best practices for state management in Angular applications, such as keeping the state immutable and using pure functions to update the state, you can ensure that your application remains performant and scalable.
Managing authentication state in Angular applications can be simplified by using Redux, as you can store the user authentication information in the Redux store and easily access it from any component in your application. By using services vs. NgRx for state management in Angular, you can choose the approach that best fits the needs of your application and team.
Overall, integrating Redux with Angular can help you optimize performance with efficient state management, handle complex state transitions, and manage global vs. local state in your Angular applications. By following state management patterns for Angular components and leveraging the power of Redux, you can create a more robust and maintainable application.Redux DevTools for Angular
Redux DevTools for Angular is a powerful tool that allows Angular programmers to inspect and debug their application’s state in real-time. By integrating Redux DevTools into your Angular project, you can gain valuable insights into how your application’s state changes over time, making it easier to identify and fix bugs.
One of the key benefits of using Redux DevTools in Angular is the ability to track state changes through a time-traveling debugger. This feature allows you to rewind and replay state changes, making it easier to pinpoint the source of any issues that may arise in your application. Additionally, Redux DevTools provides a visual representation of your application’s state, making it easier to understand complex state structures and relationships.
In addition to debugging, Redux DevTools also offers a range of other useful features for Angular programmers. For example, you can use the tool to monitor performance metrics, such as action dispatch times and state update times, helping you identify potential bottlenecks in your application’s state management.
Overall, integrating Redux DevTools into your Angular project can greatly improve your ability to manage state effectively and efficiently. By providing powerful debugging tools and performance monitoring capabilities, Redux DevTools empowers Angular programmers to build robust and high-performing applications with ease. If you are looking to take your state management skills to the next level, Redux DevTools for Angular is a must-have tool in your toolkit.
Chapter 5: Managing Authentication State in Angular Applications
Authentication State Management
Authentication State Management is a crucial aspect of state management in Angular applications. It is essential for ensuring that only authenticated users can access certain parts of the application and perform specific actions.
One of the best practices for managing authentication state in Angular is to use services to handle user authentication and authorization. By creating a service specifically for managing user authentication, you can encapsulate all the logic related to user login, logout, and access control in one place. This makes it easier to maintain and update the authentication logic as needed.
Another popular approach to authentication state management in Angular is to use NgRx, a powerful state management library inspired by Redux. NgRx allows you to define a centralized store for managing the application state, including the authentication state. By dispatching actions and handling them with reducers, you can easily update the authentication state in response to user actions.
Implementing Redux in Angular for state management is another effective way to manage authentication state. By following the principles of Redux, you can create a single source of truth for the application state, making it easier to manage complex state transitions and ensure consistency across different parts of the application.
When it comes to handling complex state transitions in Angular applications, RxJS can be a valuable tool. By using observables and operators provided by RxJS, you can easily manage asynchronous data streams and handle complex state changes in a reactive and efficient way.
In conclusion, managing authentication state in Angular applications requires careful planning and implementation. By following best practices, using the right tools and libraries, and adopting efficient state management patterns, you can ensure a secure and seamless user experience in your Angular applications.Securing Angular Applications
As an Angular programmer, one of the key aspects of developing robust applications is ensuring that they are secure. Securing Angular applications involves not only protecting sensitive data but also safeguarding against potential threats such as cross-site scripting (XSS) attacks and security vulnerabilities.
When it comes to state management in Angular applications, security should be a top priority. Best practices for state management in Angular applications include encrypting sensitive data, implementing proper authentication mechanisms, and following secure coding practices.
One popular approach to state management in Angular is using NgRx, which is a powerful Redux-inspired state management library. By implementing Redux in Angular, developers can ensure a centralized and predictable state management system that makes it easier to track and debug application state.
Managing authentication state in Angular applications is crucial for ensuring that only authorized users have access to sensitive data and features. Implementing secure authentication mechanisms, such as token-based authentication, can help prevent unauthorized access to protected resources.
State management with RxJS in Angular is another effective way to handle complex state transitions in applications. By using observables and operators provided by RxJS, developers can create reactive and efficient state management systems that respond to changes in real-time.
When it comes to choosing between using services or NgRx for state management in Angular, developers should consider factors such as the complexity of the application, scalability requirements, and performance considerations. While services are suitable for managing local state within components, NgRx is more suitable for managing global state across the application.
Optimizing performance with efficient state management in Angular involves minimizing unnecessary state updates, using memoization techniques, and leveraging lazy loading strategies. By implementing efficient state management practices, developers can improve the overall performance and user experience of Angular applications.
In conclusion, securing Angular applications involves implementing best practices for state management, utilizing tools like NgRx and RxJS, and optimizing performance for efficient state management. By following these guidelines, Angular programmers can develop secure and robust applications that meet the highest standards of security and performance.Implementing Authentication with NgRx
In any modern web application, authentication is a crucial aspect that ensures the security and privacy of users’ data. With Angular, managing authentication state can become complex as the application grows in size and complexity. This is where NgRx, a powerful state management library inspired by Redux, comes into play.
NgRx provides a centralized store to manage the state of your application, including authentication state. By implementing authentication with NgRx, you can ensure a single source of truth for the authentication status of the user across your application.
To implement authentication with NgRx, you first need to define the necessary actions, reducers, selectors, and effects. Actions represent the events that can change the authentication state, such as logging in or logging out. Reducers handle these actions and update the authentication state in the store. Selectors allow you to access the authentication state from different components in a consistent and predictable way. Effects enable you to perform side effects, such as making HTTP requests to authenticate the user.
By using NgRx for authentication state management, you can ensure that the authentication logic is separated from the components, making your codebase more maintainable and testable. Additionally, NgRx’s immutable state management ensures that changes to the authentication state are predictable and traceable.
In conclusion, implementing authentication with NgRx in your Angular application can streamline the management of user authentication state and improve the overall security and reliability of your application. By following best practices for state management and leveraging the power of NgRx, you can create a robust and scalable authentication system for your Angular application.
Chapter 6: State Management with RxJS in Angular
RxJS Basics
RxJS (Reactive Extensions for JavaScript) is a powerful library that provides a set of tools for managing asynchronous data streams within Angular applications. Understanding the basics of RxJS is essential for effective state management in Angular.
At its core, RxJS enables Angular programmers to work with observables, which are data streams that can be manipulated, transformed, and combined in various ways. By utilizing observables, developers can create a reactive programming style that allows for seamless state management and data flow within their applications.
One key concept in RxJS is the use of operators, which are functions that can be applied to observables to perform operations such as filtering, mapping, and reducing data streams. By using operators effectively, Angular programmers can manipulate data streams in a declarative and composable manner, making their code more readable and maintainable.
When it comes to state management in Angular applications, RxJS can be used in conjunction with other tools such as NgRx and Redux to implement robust state management solutions. By leveraging the power of observables and operators, developers can create reactive data flows that update the application state in a predictable and efficient manner.
In addition to managing application state, RxJS can also be used to handle complex state transitions, optimize performance, and manage global vs. local state within Angular applications. By understanding the various state management patterns and best practices for using RxJS in Angular, developers can build scalable and maintainable applications that meet the needs of their users.
In conclusion, RxJS is a valuable tool for Angular programmers looking to implement effective state management strategies in their applications. By mastering the basics of RxJS and incorporating it into their development workflow, developers can create robust and efficient state management solutions that enhance the user experience and streamline their codebase.Observables and Subjects
Observables and Subjects are important concepts to understand when it comes to state management in Angular applications. Observables are a key part of the RxJS library, which is commonly used for handling asynchronous data streams in Angular. They allow us to subscribe to a stream of data and react to any changes that occur over time.
Subjects are a type of Observable that allow us to both publish values to a stream and subscribe to those values. This makes them particularly useful for managing state in our Angular applications. By using Subjects, we can create a centralized store of state that can be accessed and updated from various parts of our application.
When it comes to state management in Angular, it’s important to follow best practices to ensure our code is maintainable and scalable. One popular approach is to use NgRx, which is a Redux-inspired state management library for Angular. NgRx provides a set of tools and patterns for managing state in a predictable way, making it easier to debug and test our applications.
Implementing Redux in Angular can help us keep our application’s state in sync and make it easier to reason about the flow of data. By using actions, reducers, and selectors, we can ensure that state changes are handled consistently throughout our application.
Managing authentication state in Angular applications is another important consideration. By using services or NgRx to manage the authentication state, we can ensure that users are properly authenticated and authorized to access certain parts of our application.
In conclusion, Observables and Subjects are powerful tools for state management in Angular applications. By following best practices, using NgRx, and implementing Redux, we can effectively manage state in our applications and optimize performance for a better user experience.Combining RxJS with Angular State Management
As an Angular programmer, you may already be familiar with the concept of state management in Angular applications. State management is crucial for maintaining the integrity and consistency of your application’s data throughout its lifecycle. One popular approach to state management in Angular is using RxJS, a reactive programming library that allows you to work with asynchronous data streams.
By combining RxJS with Angular’s built-in state management capabilities, you can create a robust and efficient state management system for your application. RxJS provides powerful tools for handling complex state transitions, managing global and local state, and optimizing performance through efficient data handling.
One key benefit of using RxJS for state management in Angular is its ability to handle asynchronous data streams in a reactive and declarative manner. This allows you to easily manage complex state transitions and handle data flow in a more predictable and manageable way.
In addition, RxJS can be used to create observables that represent different pieces of your application’s state. These observables can be subscribed to in various components of your application, allowing for easy sharing and synchronization of state across different parts of your application.
When combined with Angular’s component-based architecture, RxJS can help you implement a more efficient and scalable state management system for your application. By using observables and operators provided by RxJS, you can easily manage authentication state, implement Redux-like patterns, and optimize performance by handling data streams more effectively.
In conclusion, combining RxJS with Angular’s state management capabilities can provide you with a powerful and flexible toolset for managing state in your Angular applications. By leveraging the reactive and declarative nature of RxJS, you can create a more robust and efficient state management system that meets the needs of your application.
Chapter 7: Handling Complex State Transitions in Angular Applications
Asynchronous State Updates
In the world of Angular programming, managing state in an application is crucial for ensuring a seamless user experience. One of the challenges that developers often face is handling asynchronous state updates, where data changes may occur at unpredictable times and need to be reflected in the application’s user interface.
When it comes to dealing with asynchronous state updates in Angular, there are several best practices that developers can follow. One popular approach is to use NgRx, a powerful state management library that implements the Redux pattern. By centralizing application state and using actions and reducers to update it in a predictable way, NgRx provides a robust solution for managing asynchronous state updates in Angular applications.
Another option for implementing asynchronous state updates in Angular is to use RxJS, a library for reactive programming that provides a range of operators for working with asynchronous data streams. By using observables and operators such as mergeMap and switchMap, developers can handle complex state transitions and manage asynchronous updates in a more efficient and scalable way.
In addition to using NgRx and RxJS, developers can also consider other state management patterns for Angular components, such as using services to encapsulate and manage state within individual components. While NgRx provides a centralized approach to state management, using services can be a more lightweight alternative for managing local state within components.
Overall, when it comes to handling asynchronous state updates in Angular applications, developers have a range of tools and strategies at their disposal. By following best practices, leveraging libraries like NgRx and RxJS, and choosing the right state management patterns for their specific needs, Angular programmers can ensure that their applications are both performant and responsive to user interactions.Error Handling in State Management
Error handling is an essential aspect of state management in Angular applications. As an Angular programmer, it is important to have a robust error handling mechanism in place to ensure that your application runs smoothly and efficiently.
When it comes to error handling in state management, there are a few best practices that you should keep in mind. First and foremost, it is important to have a clear understanding of the different types of errors that can occur in your application. By identifying potential error scenarios, you can proactively implement error handling mechanisms to address them.
One popular approach to error handling in Angular applications is to use NgRx for state management. NgRx provides a powerful and flexible way to manage state in Angular applications, and it includes built-in error handling capabilities that make it easy to handle errors gracefully.
Another effective way to handle errors in state management is to implement Redux in Angular. Redux is a predictable state container that helps you manage the state of your application in a consistent and reliable manner. By using Redux, you can easily handle errors and ensure that your application remains stable and responsive.
When it comes to managing authentication state in Angular applications, it is important to have a robust error handling mechanism in place to handle authentication errors effectively. By implementing error handling strategies, you can ensure that your application remains secure and user-friendly.
In conclusion, error handling is a critical aspect of state management in Angular applications. By following best practices and using tools like NgRx and Redux, you can effectively handle errors and ensure that your application runs smoothly and efficiently.Optimistic Updates
In the world of Angular development, staying up to date with the latest trends and best practices is crucial for success. As an Angular programmer, you need to be constantly striving to optimize your state management strategies in order to create efficient and high-performing applications.
One of the keys to successful state management in Angular is staying optimistic in your updates. Optimistic updates involve updating the client-side UI before the server-side response is received, giving the user instant feedback and creating a more responsive user experience. This can be especially useful in scenarios where network latency may slow down the process of updating data.
To implement optimistic updates in your Angular application, you can use techniques such as local state management with RxJS or NgRx. By storing state locally and updating the UI immediately, you can improve the perceived performance of your application and create a more seamless user experience.
When managing authentication state in Angular applications, optimistic updates can also be beneficial. By instantly updating the UI to reflect a successful login attempt, you can give users immediate feedback and reduce frustration.
In order to effectively handle complex state transitions in Angular applications, using optimistic updates can simplify the process and make your code more maintainable. By breaking down state management into smaller, more manageable pieces, you can create a more organized and efficient application.
Overall, staying optimistic in your updates is a key strategy for successful state management in Angular. By implementing optimistic updates in your application, you can improve performance, create a more responsive user experience, and simplify complex state transitions. So, keep an open mind and stay optimistic in your state management strategies for Angular development.
Chapter 8: Using Services vs. NgRx for State Management in Angular
Pros and Cons of Services
State management is a crucial aspect of developing robust and efficient Angular applications. One key component of state management in Angular is the use of services. Services are a common way to centralize and manage application state, but they come with their own set of pros and cons.
One of the main advantages of using services for state management in Angular is their simplicity and ease of use. Services allow for easy sharing of data between components and provide a centralized location for managing application state. This can make it easier to maintain and update state across different parts of the application.
Another advantage of using services for state management is their flexibility. Services can be easily injected into different components, making it simple to access and update state from anywhere in the application. This can help to reduce code duplication and make it easier to maintain a clean and organized codebase.
However, there are also some drawbacks to using services for state management in Angular. One potential downside is the risk of creating a complex and difficult-to-maintain state management system. As the application grows and more features are added, it can be easy for services to become bloated and difficult to manage.
Another potential drawback of using services for state management is the lack of built-in tools for handling complex state transitions. Services alone may not provide the necessary tools for managing asynchronous data flows or handling complex state changes.
In conclusion, services can be a valuable tool for state management in Angular applications, but they may not always be the best solution for every situation. It is important to carefully weigh the pros and cons of using services for state management and consider other options, such as NgRx or Redux, depending on the specific needs of the application.Pros and Cons of NgRx
NgRx is a popular state management library for Angular applications that is based on the Redux pattern. It provides a centralized store for managing the state of an application and enables developers to easily manage complex state transitions. However, like any tool, NgRx comes with its own set of pros and cons that developers should consider before using it in their projects.
One of the main advantages of NgRx is that it enforces a strict and predictable state management pattern, which can help to organize code and make it more maintainable. By using NgRx, developers can easily track state changes and debug issues, as all state mutations are handled through actions and reducers.
Additionally, NgRx provides powerful tools for handling asynchronous operations, such as the use of effects to manage side effects like HTTP requests. This can help to simplify code and prevent common pitfalls associated with managing asynchronous data in Angular applications.
On the other hand, using NgRx can also introduce complexity to an application, especially for developers who are not familiar with the Redux pattern. The boilerplate code required to set up actions, reducers, and selectors can be overwhelming for beginners and may slow down development time.
Furthermore, NgRx can also lead to over-engineering in some cases, as developers may be tempted to use it for simple state management tasks that could be handled more efficiently with Angular services. This can result in bloated code and unnecessary complexity.
In conclusion, NgRx is a powerful tool for managing state in Angular applications, but developers should carefully weigh the pros and cons before deciding to use it in their projects. It is important to consider factors such as the complexity of the application, the experience level of the development team, and the specific state management requirements of the project.Choosing the Right Approach for State Management
Choosing the right approach for state management in an Angular application is crucial for maintaining a clean and efficient codebase. There are various strategies and tools available for managing state in Angular, each with its own pros and cons. As an Angular programmer, it is important to understand these approaches and choose the one that best fits the requirements of your project.
One common approach to state management in Angular is using services. Services are a great way to encapsulate stateful logic and share data between components. They are simple to implement and can be easily injected into components. However, services can become cumbersome to manage as the application grows in complexity.
Another popular approach is using NgRx, a state management library inspired by Redux. NgRx provides a centralized store for managing state and allows for predictable state transitions using actions and reducers. While NgRx can be powerful for managing complex state in large applications, it comes with a steep learning curve and boilerplate code.
When it comes to managing authentication state in Angular applications, using services or NgRx can both be viable options. Services can be used to store authentication tokens and user information, while NgRx can be used for handling complex authentication workflows.
For handling complex state transitions in Angular applications, RxJS can be a powerful tool. By using observables and operators, you can create reactive data streams that can be easily manipulated and transformed to manage state changes.
Ultimately, the best approach for state management in an Angular application will depend on the specific requirements and constraints of your project. It is important to evaluate the trade-offs of each approach and choose the one that best aligns with your project goals and development team’s expertise. By carefully considering these factors, you can ensure that your Angular application has a robust and efficient state management system in place.
Chapter 9: Optimizing Performance with Efficient State Management in Angular
Performance Considerations in State Management
Performance considerations in state management are crucial for ensuring that your Angular application runs smoothly and efficiently. When managing state in an Angular application, there are several best practices that can help optimize performance and improve the overall user experience.
One key consideration is the use of NgRx for state management in Angular. NgRx is a powerful library that implements the Redux pattern for managing application state. By using NgRx, you can centralize your application state and ensure that changes are handled in a predictable and efficient manner.
Another important aspect of performance optimization in state management is implementing Redux in Angular. Redux is a popular state management pattern that emphasizes immutability and unidirectional data flow. By following the principles of Redux, you can create a more maintainable and performant Angular application.
Managing authentication state is another critical consideration for performance in Angular applications. By efficiently managing authentication state, you can ensure that users are authenticated only when necessary and minimize unnecessary API calls.
State management with RxJS is also a powerful tool for optimizing performance in Angular applications. By using RxJS observables, you can handle complex state transitions and asynchronous operations in a more efficient manner.
In conclusion, optimizing performance with efficient state management in Angular involves using the right tools and techniques to ensure that your application runs smoothly and responsively. By following best practices and leveraging tools like NgRx, Redux, and RxJS, you can create a high-performing Angular application that provides a seamless user experience.Memoization and Selectors
Memoization and Selectors are key concepts in state management for Angular applications. Memoization is a technique used to optimize performance by storing the results of expensive function calls and returning the cached result when the same inputs occur again. This can be particularly useful when dealing with complex state transitions or computations in your application.
Selectors, on the other hand, are functions that extract specific pieces of state from your application’s store. They allow you to efficiently access and manipulate data without directly accessing the store itself. Selectors can help simplify your code and improve the maintainability of your application by encapsulating the logic for accessing specific pieces of state.
When implementing state management in Angular, it’s important to consider the best practices for managing state in your application. Using NgRx, a powerful library for managing state in Angular applications, can help streamline the process and ensure that your state management is efficient and scalable.
Implementing Redux in Angular is another popular approach for managing state in Angular applications. Redux provides a predictable state container that can help simplify the management of complex state transitions and data flow in your application.
When managing authentication state in Angular applications, it’s important to carefully consider how you handle user authentication and authorization. Using state management techniques such as NgRx or RxJS can help ensure that your authentication state is secure and efficiently managed.
Overall, state management in Angular applications can be complex, but by utilizing techniques such as memoization, selectors, and libraries like NgRx and Redux, you can optimize performance, improve code maintainability, and ensure that your application’s state is efficiently managed. By carefully considering the best practices for state management and choosing the right tools for your application, you can create a robust and reliable state management system for your Angular projects.Lazy Loading State Modules
Lazy loading state modules is a crucial aspect of state management in Angular applications. As an Angular programmer, it is important to understand how to efficiently load state modules to improve the performance and user experience of your application.
Lazy loading state modules involves loading modules only when they are needed, rather than loading all modules at once when the application is first loaded. This can help reduce the initial load time of your application and improve the overall performance by only loading the necessary modules when they are required.
One of the best practices for state management in Angular applications is to use lazy loading for state modules. By lazy loading state modules, you can improve the performance of your application and ensure that only the necessary modules are loaded at any given time.
NgRx is a popular library for state management in Angular applications, and it provides support for lazy loading state modules. By implementing lazy loading with NgRx, you can efficiently manage the state of your application and improve the overall performance.
When using NgRx for state management in Angular, it is important to consider the implementation of lazy loading state modules to optimize the performance of your application. By lazy loading state modules, you can reduce the initial load time and improve the user experience.
In conclusion, lazy loading state modules is an important strategy for efficient state management in Angular applications. By implementing lazy loading with NgRx or other state management libraries, you can optimize the performance of your application and provide a better user experience for your users.
Chapter 10: Managing Global vs. Local State in Angular Applications
Global State Management
State management is a crucial aspect of building robust and efficient Angular applications. In order to ensure that your application runs smoothly and maintains a consistent state across different components, it is important to implement effective global state management strategies.
Global state management involves managing the state of your application at a higher level, allowing you to share data and communicate between different components without having to pass data down through a complex hierarchy of parent and child components. By centralizing your application’s state in a global store, you can simplify the process of updating and accessing data, making your code more maintainable and scalable.
One of the best practices for state management in Angular applications is to use NgRx, a powerful state management library inspired by Redux. NgRx allows you to define a single source of truth for your application’s state, and provides tools for managing complex state transitions and side effects. By following the principles of immutable state and unidirectional data flow, you can ensure that your application remains predictable and easy to debug.
When implementing Redux in Angular for state management, you can use concepts such as actions, reducers, and selectors to update and retrieve data from your global store. By organizing your state management logic into separate modules, you can keep your codebase clean and maintainable, making it easier to add new features and scale your application.
Managing authentication state in Angular applications is another important aspect of global state management. By storing user authentication information in a global store, you can easily check if a user is logged in and enforce access control policies throughout your application. By using RxJS to handle asynchronous operations and side effects, you can ensure that your authentication state remains consistent and up-to-date.
In order to optimize performance with efficient state management in Angular, it is important to carefully design your state management architecture and avoid unnecessary re-renders and data fetches. By using services to manage local state within individual components, you can reduce the complexity of your global state and improve the performance of your application. By following best practices for managing global vs. local state in Angular applications, you can strike a balance between centralization and decentralization, ensuring that your application remains responsive and scalable.
Overall, state management is a key aspect of building maintainable and efficient Angular applications. By following best practices and using tools such as NgRx and Redux, you can simplify the process of managing complex state transitions and ensure that your application remains predictable and performant. By optimizing your state management strategies and following established patterns for managing state in Angular components, you can build high-quality applications that meet the needs of your users and stakeholders.Local Component State Management
Local Component State Management is a crucial aspect of building robust and efficient Angular applications. In this subchapter, we will discuss the best practices for managing state at the component level, which plays a significant role in ensuring a seamless user experience.
When it comes to managing state in an Angular application, developers often face the dilemma of choosing between using services or NgRx. While NgRx offers a centralized and scalable approach to state management, it may be overkill for managing local component state. In such cases, using services to handle component-specific state can be more efficient and straightforward.
Implementing Redux in Angular for state management is another powerful strategy that can help in maintaining a single source of truth for the application state. By following the principles of Redux, such as immutability and pure functions, developers can ensure predictable state transitions and easier debugging.
Managing authentication state is a critical aspect of many Angular applications. By leveraging local component state management techniques, developers can efficiently handle authentication-related logic within individual components without cluttering the global state.
State management with RxJS in Angular is another popular approach that allows developers to handle complex state transitions using reactive programming principles. By leveraging observables, developers can create responsive and scalable applications that react to changes in state seamlessly.
In conclusion, understanding the various state management patterns for Angular components and choosing the right strategy based on the specific requirements of the application is essential for building performant and maintainable Angular applications. By optimizing performance with efficient state management techniques and striking the right balance between global and local state management, developers can create robust and responsive applications that meet the needs of their users effectively.Sharing State between Components
Sharing state between components is a crucial aspect of managing state in an Angular application. By enabling communication between different components, you can ensure that your application remains consistent and responsive to user interactions. There are several strategies and best practices that Angular programmers can follow to effectively share state between components.
One popular approach to sharing state in Angular applications is using NgRx, which is a powerful state management library inspired by Redux. NgRx allows you to define a single source of truth for your application state and provides mechanisms for updating and accessing this state from different components. By implementing Redux principles in Angular, you can ensure a predictable state management flow and simplify the debugging process.
Another important consideration when sharing state between components is managing authentication state. It is essential to securely store and update user authentication information across different parts of your application. By centralizing authentication state management, you can ensure that user sessions remain consistent and secure throughout the application.
In addition to using NgRx, Angular programmers can leverage the power of RxJS for handling complex state transitions. RxJS provides a set of operators and observables that enable reactive programming and facilitate communication between components in a seamless manner. By using observables to represent state changes, you can create a more responsive and efficient application.
When deciding between using services or NgRx for state management in Angular, it is essential to consider the performance implications of each approach. While services are a lightweight and straightforward way to share state between components, NgRx provides a more structured and scalable solution for managing complex application state.
In conclusion, sharing state between components is a critical aspect of state management in Angular applications. By following best practices and utilizing tools like NgRx and RxJS, Angular programmers can ensure that their applications are efficient, responsive, and maintainable.
Chapter 11: State Management Patterns for Angular Components
Container and Presentation Components
In Angular applications, state management is a crucial aspect of development that can greatly impact the performance and user experience of the application. One key consideration when it comes to state management is how to handle container and presentation components in your application.
Container components are responsible for managing the state of the application and passing data down to presentation components. They are typically connected to the store or service that holds the application’s state and are responsible for fetching and updating data as needed. Presentation components, on the other hand, are responsible for rendering the UI based on the data provided to them by the container components.
When designing your Angular application, it’s important to follow best practices for state management to ensure that your application is scalable, maintainable, and performant. One popular approach to state management in Angular is to use NgRx, a Redux-inspired library that provides a centralized store for managing application state. By implementing NgRx in your application, you can easily manage complex state transitions, optimize performance, and handle authentication state effectively.
Alternatively, you can also implement Redux directly in your Angular application to manage state. Redux provides a predictable state container that can help simplify state management and make it easier to debug and test your application.
When it comes to handling state management in Angular, you may also need to consider whether to use services or NgRx for managing state. While services can be a simple and effective way to manage state in smaller applications, NgRx can provide more advanced features and optimizations for larger and more complex applications.
Overall, managing state in Angular applications requires careful consideration of how to structure your components, whether to use NgRx or Redux, and how to optimize performance. By following best practices and choosing the right approach for your application, you can ensure that your Angular application is well-structured, efficient, and user-friendly.Smart and Dumb Components
In the world of Angular development, understanding the concept of smart and dumb components is essential for effective state management. Smart components, also known as container components, are responsible for managing the state of an application. They interact with services, fetch data from APIs, and pass this data down to dumb components for rendering.
On the other hand, dumb components, also known as presentational components, are purely focused on presenting data. They receive data from smart components as input through @Input bindings and emit events to smart components through @Output bindings.
When it comes to state management in Angular applications, it is crucial to strike a balance between smart and dumb components. Smart components should handle complex state transitions, manage authentication state, and make use of libraries like NgRx or implement the Redux pattern for efficient state management.
Dumb components, on the other hand, should be kept as simple and reusable as possible. They should not have any knowledge of the application’s state and should only focus on rendering the data provided to them.
By following best practices for state management in Angular applications, such as using RxJS for handling asynchronous operations and optimizing performance by efficiently managing state, developers can create scalable and maintainable applications.
Whether you choose to use services or NgRx for state management in Angular, it is important to consider the specific requirements of your application and choose the approach that best fits your needs. By implementing state management patterns for Angular components and carefully managing global vs. local state, developers can create robust and efficient applications that provide a seamless user experience.Stateful and Stateless Components
In Angular development, understanding the concepts of stateful and stateless components is crucial for effective state management. Stateful components are those that have their own internal state, meaning they can change and store data throughout their lifecycle. On the other hand, stateless components do not have any internal state and rely solely on input properties to display data.
When it comes to managing state in an Angular application, it is important to strike a balance between stateful and stateless components. Stateful components are useful for handling local state specific to a particular component, while stateless components are great for displaying data and maintaining a clean and predictable component hierarchy.
Best practices for state management in Angular applications involve using services for managing shared state and business logic, while keeping components as dumb and reusable as possible. This separation of concerns helps in maintaining a scalable and maintainable codebase.
One popular library for state management in Angular is NgRx, which implements the Redux pattern to manage application state in a predictable and centralized way. By using NgRx, developers can easily handle complex state transitions, manage authentication state, and optimize performance through efficient state management using RxJS.
When it comes to managing global versus local state in Angular applications, it is important to carefully analyze the requirements of each component and decide whether using services or NgRx is the best approach. While services are great for managing local state, NgRx provides a robust solution for managing global state across multiple components.
In conclusion, understanding the concepts of stateful and stateless components, implementing Redux with NgRx, and utilizing best practices for state management in Angular can greatly enhance the efficiency and scalability of your application. By following these guidelines, Angular programmers can effectively manage state in their applications and build robust and maintainable codebases.
1 thought on “How do you manage state in an Angular application?”