Skip to content
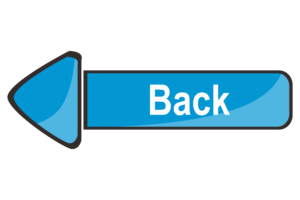
Describe how to create and use interceptors in Angular:
1: What are interceptors?
- Interceptors are a mechanism in Angular that allows us to intercept and manipulate HTTP requests and responses.
- They can be used to perform common tasks such as adding headers, logging, error handling, etc.
2: Creating an interceptor
- To create an interceptor, we need to implement the
HttpInterceptor
interface.
- This interface provides two methods:
intercept()
and handle()
.
3:The intercept()
method
- The
intercept()
method is responsible for intercepting the HTTP requests and adding any necessary headers, or performing other operations.
- It takes two parameters:
req
, which represents the original request, and next
, which is an instance of the HttpHandler
class.
- The
intercept()
method needs to return an Observable of the HttpRequest or an HttpEvent.
4:The handle()
method
- The
handle()
method is used to forward the request or response through the interceptor chain.
- It takes an instance of the
HttpRequest
class as a parameter and returns an Observable of the HttpEvent
.
5:Registering the interceptor
- Once the interceptor is created, we need to register it in the application.
- We can do this by providing it in the
HTTP_INTERCEPTORS
token of the Angular’s dependency injection system.
- We can also specify the order in which the interceptors should be executed by providing a multi-provider with a specific
providedIn
value.
6:Using interceptors
- Once the interceptor is registered, it will be automatically applied to all HTTP requests and responses.
- The interceptors can be used to modify the requests or responses as needed.
- For example, adding a token to the request headers or handling authentication errors.
7:Multiple interceptors
- Angular allows us to have multiple interceptors in the application.
- The order of execution of the interceptors can be controlled by specifying the order in which they are registered.
- The interceptors will be executed in the order of their registration.
8:Advanced usage
- Interceptors can also be used for other advanced purposes like caching, request throttling, etc.
- We can leverage interceptors to implement complex logic and enhance the application’s performance and functionality.
9:Conclusion
- Intercepters in Angular provide a powerful mechanism to intercept and manipulate HTTP requests and responses.
- We can create interceptors, register them in the application, and use them to modify requests or responses as needed.
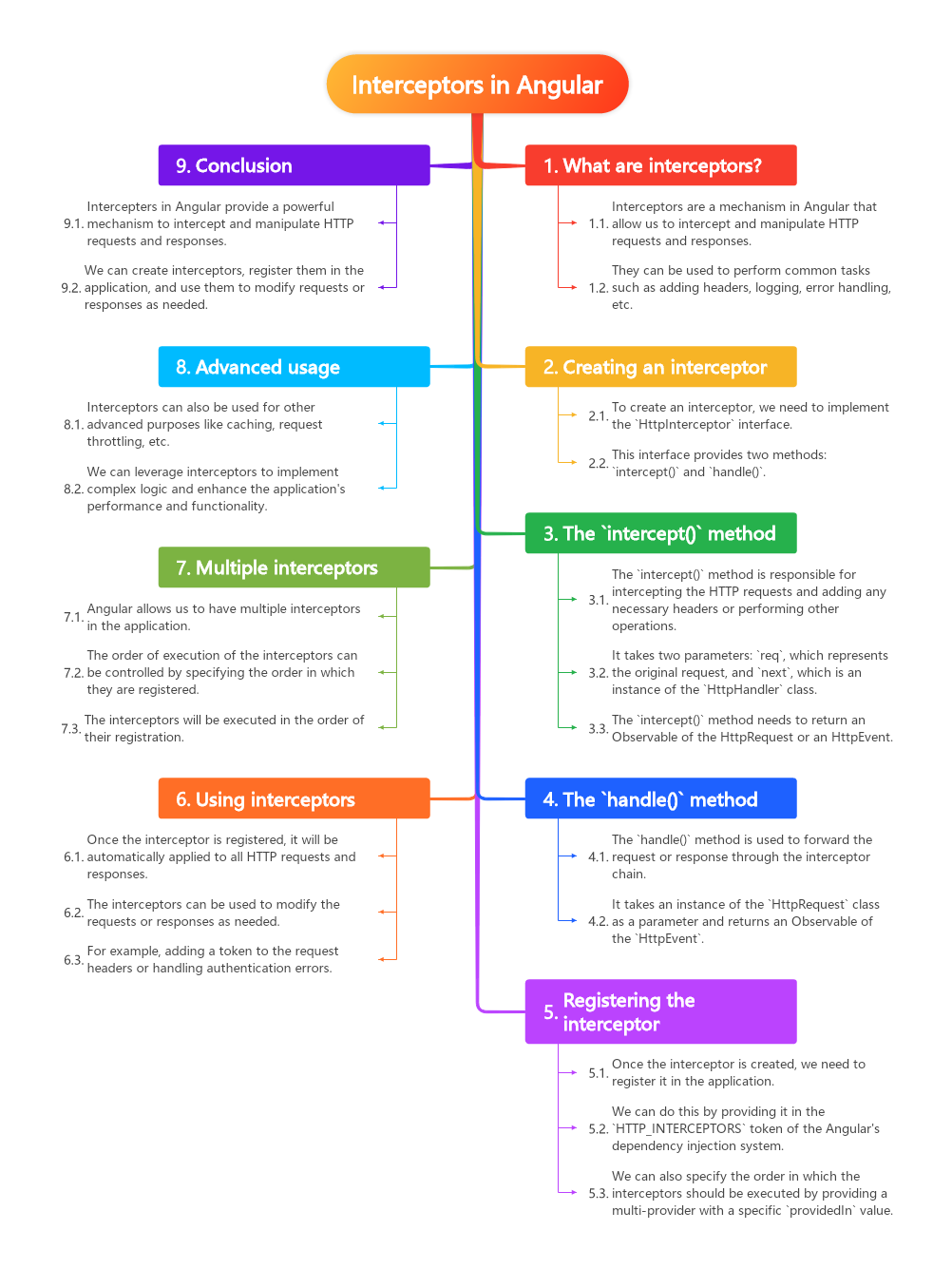
1 thought on “Describe How To Create And Use Interceptors In Angular”