What are guards in Angular routing and how are they used?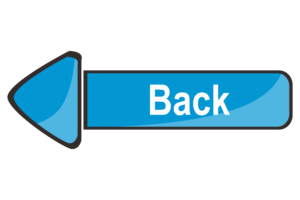
1: Introduction to Angular Routing Guards
Understanding Angular Routing
Angular routing is a crucial aspect of building web applications using Angular. It allows us to navigate between different components of our application seamlessly and efficiently. However, in order to control the navigation flow and access to certain routes, Angular provides us with the concept of guards. Understanding Angular routing guards is essential for any Angular programmer looking to build robust and secure applications.
Guards in Angular routing are used to protect routes in our application by implementing specific logic before allowing access to a particular route. There are several types of guards available in Angular, including CanActivate, CanActivateChild, CanDeactivate, and CanLoad. Each guard serves a different purpose and can be used to control access to routes based on various conditions.
The CanActivate guard is used to determine whether a user can access a specific route. It is commonly used to check if a user is authenticated before allowing access to a protected route. CanActivateChild, on the other hand, is similar to CanActivate but applies to child routes of a parent route. CanDeactivate is used to determine if a user can leave a route, while CanLoad is used to prevent a module from being loaded lazily.
Implementing guards in Angular routing involves creating a guard service that implements the necessary logic for each guard type. These guard services are then added to the route configuration using the canActivate, canActivateChild, canDeactivate, or canLoad properties. By using guards, Angular programmers can control access to routes, protect sensitive information, and enhance the overall security of their applications.
In conclusion, understanding Angular routing guards is crucial for building secure and efficient web applications using Angular. By leveraging guards such as CanActivate, CanActivateChild, CanDeactivate, and CanLoad, Angular programmers can control access to routes, protect sensitive information, and enhance the overall security of their applications. With the knowledge of Angular routing guards, programmers can build robust and secure applications that provide a seamless user experience.
What are Guards in Angular Routing?
Guards in Angular routing serve as a crucial mechanism for controlling access to certain routes within an Angular application. They act as filters that determine whether a user is authorized to navigate to a particular route or not. In essence, guards help in enforcing security and access control in an Angular application by providing a way to protect certain routes from unauthorized access.
There are several types of guards in Angular routing, namely CanActivate, CanActivateChild, CanDeactivate, and CanLoad. Each guard type serves a specific purpose and can be used to enforce different types of access control rules within an application. CanActivate is used to determine whether a user can access a particular route, CanActivateChild is used to determine access to child routes of a route, CanDeactivate is used to determine whether a user can leave a route, and CanLoad is used to determine whether a user can load a module lazily.
Guards are implemented as services in Angular and can be attached to routes using the canActivate, canActivateChild, canDeactivate, and canLoad properties in the route configuration. When a user attempts to navigate to a route that is protected by a guard, the guard is invoked and its logic is executed to determine whether the user is authorized to access the route. If the guard returns true, the user is allowed to navigate to the route. If the guard returns false or a Promise or Observable that resolves to false, the navigation is canceled, and the user is redirected to a different route.
Guards in Angular routing play a crucial role in ensuring the security and integrity of an application by controlling access to certain routes based on predefined rules and conditions. By using guards effectively, Angular programmers can enforce access control, prevent unauthorized access to sensitive routes, and provide a secure and seamless user experience within their applications. In the subsequent chapters of this book, we will delve deeper into each type of guard, discuss best practices for implementing guards, and explore advanced use cases for guards in Angular routing.
2: Types of Angular Routing Guards
CanActivate Guard
In Angular, guards are used to control access to certain routes in your application. One type of guard that can be used is the CanActivate guard. This guard is used to determine whether a user can access a certain route based on certain conditions.
The CanActivate guard is implemented as a service that implements the CanActivate interface. This interface has a single method called canActivate, which returns a boolean value indicating whether the route can be activated. If the method returns true, the route is activated and the user can access it. If the method returns false, the route is not activated and the user is redirected to another route.
One common use case for the CanActivate guard is to prevent unauthorized users from accessing certain routes in your application. For example, you can create a guard that checks whether the user is logged in before allowing them to access the dashboard route. If the user is not logged in, the guard can redirect them to the login page instead.
Another use case for the CanActivate guard is to prevent users from accessing certain routes based on their role or permissions. For example, you can create a guard that checks whether the user is an admin before allowing them to access the admin dashboard route. If the user is not an admin, the guard can redirect them to another route or display an error message.
Overall, the CanActivate guard is a powerful tool that can be used to control access to routes in your Angular application. By implementing guards like CanActivate, you can ensure that your application is secure and that users can only access the routes that they are authorized to access.
CanDeactivate Guard
In Angular, guards are used to protect and control access to different parts of an application based on certain conditions. One such guard is the CanDeactivate guard, which is used to prevent users from navigating away from a particular route without confirming their action. This guard is commonly used in forms or other components where unsaved changes could be lost if the user navigates away without saving.
The CanDeactivate guard works by implementing a CanDeactivate interface in the component that needs protection. This interface requires the component to have a canDeactivate method that returns a boolean or a Promise. If the method returns true, the navigation is allowed, but if it returns false, the user is prompted to confirm their action before leaving the page.
To implement the CanDeactivate guard in Angular, you first need to create a guard service that implements the CanDeactivate interface. This service will be responsible for checking if the canDeactivate method in the component returns true or false. If it returns false, the guard service can display a confirmation dialog to the user asking if they are sure they want to leave the page.
Once the guard service is implemented, it needs to be added to the route configuration in the Angular application. This is done by adding the canDeactivate property to the route object and specifying the guard service as its value. This tells Angular to use the CanDeactivate guard to protect that particular route.
Overall, the CanDeactivate guard is a useful tool for preventing users from accidentally navigating away from a page without saving their changes. By implementing this guard in your Angular application, you can provide a better user experience and prevent data loss.
Resolve Guard
In Angular routing, guards are an essential part of managing navigation and controlling access to different routes within an application. One type of guard that is commonly used is the Resolve Guard. The Resolve Guard allows developers to retrieve data before a route is activated, ensuring that the necessary data is available for the component to render properly.
The Resolve Guard is particularly useful when working with routes that require data from a server or database before they can be displayed. By using the Resolve Guard, developers can fetch the required data and ensure that the route is only activated once the data is available. This helps to prevent errors and ensures a smoother user experience.
To implement a Resolve Guard in Angular, developers need to create a service that implements the Resolve interface. This service should contain a resolve() method that fetches the necessary data and returns it to the route. The Resolve Guard is then added to the route configuration using the resolve property, specifying which data should be resolved before the route is activated.
One of the key benefits of using a Resolve Guard is that it helps to improve the performance of an Angular application. By fetching data before a route is activated, developers can ensure that the necessary data is available when the component is rendered, reducing the need for additional network requests and improving the overall speed of the application.
In conclusion, the Resolve Guard is a powerful tool for managing data retrieval in Angular routing. By using the Resolve Guard, developers can ensure that the necessary data is available before a route is activated, improving performance and providing a better user experience. By understanding how to implement and use Resolve Guards effectively, Angular programmers can take their applications to the next level.
CanLoad Guard
In Angular, guards are used to protect routes and prevent unauthorized users from accessing certain parts of your application. One type of guard that is commonly used is the CanLoad guard. This guard is used to prevent a module from being loaded lazily if certain conditions are not met.
The CanLoad guard is implemented as a service that implements the CanLoad interface provided by Angular. This interface requires the implementation of a single method called canLoad, which returns a boolean value indicating whether or not the module can be loaded. If the method returns true, the module is loaded; if it returns false, the module is not loaded.
To use the CanLoad guard, you need to define it in the routes array of your Angular application. You can do this by adding a canActivateChild property to the route configuration object and passing an array of guards to it. The CanLoad guard should be the first guard in the array, as it will be the first one to be checked when a module is about to be loaded.
One common use case for the CanLoad guard is to check if a user is authenticated before allowing them to access a certain module. You can do this by injecting the AuthenticationService into the CanLoad guard and calling a method to check if the user is logged in. If the user is not authenticated, you can redirect them to the login page or display an error message.
Overall, the CanLoad guard is a powerful tool that can help you secure your Angular application and control access to certain parts of your application. By implementing this guard, you can ensure that only authorized users can load certain modules and protect your application from unauthorized access.
3: Implementing CanActivate Guard
Setting up CanActivate Guard
In Angular, guards are used to protect routes and control access to certain parts of an application. One type of guard is the CanActivate guard, which is used to determine if a user can access a particular route. This guard is often used to check if a user is logged in or has the necessary permissions to view a certain page.
To set up a CanActivate guard in Angular, you first need to create a new guard service using the Angular CLI. You can do this by running the command ng generate guard name-of-guard. This will create a new guard service in your project with the necessary boilerplate code.
Once you have created the guard service, you need to implement the canActivate method in the service. This method should return a boolean value indicating whether or not the user is allowed to access the route. Inside the canActivate method, you can add logic to check the user’s authentication status or permissions.
After implementing the canActivate method, you need to register the guard service in the providers array of your app module. This tells Angular to use the guard service to protect routes in your application. You can do this by adding the guard service to the providers array in the app module file.
Finally, you need to add the guard service to the canActivate property of the route you want to protect. This tells Angular to run the guard service before allowing the user to access the route. You can do this by adding the guard service to the canActivate property of the route in the app routing module file.
Overall, setting up a CanActivate guard in Angular is a straightforward process that allows you to control access to routes in your application. By following these steps, you can ensure that only authorized users are able to access certain parts of your application, enhancing the security and user experience of your Angular application.
Handling Authentication in CanActivate Guard
Authentication is a crucial aspect of web applications, especially when it comes to protecting sensitive information or features from unauthorized users. In Angular, one of the ways to handle authentication is by using guards. Guards are a feature in Angular routing that allow developers to control access to certain routes based on certain conditions being met. One type of guard that is commonly used for authentication purposes is the CanActivate guard.
The CanActivate guard is a type of guard that can be used to prevent unauthorized users from accessing certain routes in an Angular application. This guard works by implementing a canActivate method that returns either a boolean value or an observable that resolves to a boolean value. If the method returns true, the user is allowed to access the route. If it returns false, the user is redirected to another route or denied access altogether.
When it comes to handling authentication in CanActivate guards, there are a few key steps that need to be taken. First, developers need to implement a service that handles authentication logic, such as checking if a user is logged in or if they have the necessary permissions to access a certain route. This service can then be injected into the CanActivate guard to perform the necessary checks.
Next, developers need to implement the canActivate method in the CanActivate guard to call the authentication service and check if the user is authorized to access the route. If the user is authorized, the method should return true. If not, it should return false or redirect the user to another route.
Overall, handling authentication in CanActivate guards requires a combination of implementing an authentication service, injecting it into the guard, and implementing the canActivate method to perform the necessary checks. By following these steps, Angular programmers can effectively control access to routes in their applications and protect sensitive information from unauthorized users.
Redirecting with CanActivate Guard
As an Angular programmer, you are likely already familiar with the concept of guards in Angular routing. Guards are used to protect routes in your application by determining whether or not a user is allowed to access a particular route. There are several types of guards available in Angular, including CanActivate, CanActivateChild, CanDeactivate, and CanLoad. In this subchapter, we will focus on the CanActivate guard and how it can be used to redirect users to a different route if they do not have the necessary permissions to access a particular route.
The CanActivate guard is a type of guard that is used to determine whether or not a user is allowed to access a particular route. This guard is typically used to protect routes that require authentication, such as a user profile page or a dashboard. When a user tries to access a route that is protected by the CanActivate guard, the guard will run a check to see if the user is logged in or has the necessary permissions to access the route. If the check fails, the guard can redirect the user to a different route, such as a login page.
To use the CanActivate guard in your Angular application, you will need to create a guard class that implements the CanActivate interface. This class will have a canActivate method that returns a boolean value indicating whether or not the user is allowed to access the route. If the canActivate method returns true, the user will be allowed to access the route. If it returns false, the user will be redirected to a different route.
In addition to redirecting users to a different route, the CanActivate guard can also be used to display a message to the user indicating why they are being redirected. This can help provide a better user experience by giving users feedback on why they are not allowed to access a particular route. By using the CanActivate guard in your Angular application, you can ensure that only authorized users are able to access certain routes, helping to improve the security and usability of your application.
In conclusion, the CanActivate guard is a powerful tool that can be used to redirect users to a different route if they do not have the necessary permissions to access a particular route. By implementing the CanActivate guard in your Angular application, you can ensure that only authorized users are able to access certain routes, helping to improve the security and usability of your application.
4: Implementing CanDeactivate Guard
Setting up CanDeactivate Guard
In Angular, guards are used to protect routes in your application by controlling access based on certain conditions. One type of guard that can be particularly useful is the CanDeactivate guard, which allows you to prevent users from navigating away from a route if certain criteria are not met. Setting up a CanDeactivate guard involves creating a service that implements the CanDeactivate interface and then attaching this service to the route you want to protect.
To set up a CanDeactivate guard, first create a new service in your Angular application that implements the CanDeactivate interface. This interface requires you to define a canDeactivate method that takes two parameters: the component being navigated away from and the current route state. Inside this method, you can add logic to determine whether the user should be allowed to navigate away from the route.
Next, you need to attach the CanDeactivate guard to the route you want to protect. This is done by adding a canDeactivate property to the route configuration in your routing module and setting it to the name of the service you created in the previous step. This tells Angular to run the canDeactivate method in the service before allowing the user to navigate away from the route.
Once you have set up the CanDeactivate guard, you can use it to control access to certain routes in your application. For example, you could use it to prevent users from navigating away from a form page if they have unsaved changes, or to prompt them with a confirmation dialog before allowing them to leave the page.
Overall, setting up a CanDeactivate guard in your Angular application can help improve the user experience by providing a layer of protection for certain routes. By implementing this guard, you can ensure that users can only navigate away from a route if certain conditions are met, helping to prevent accidental data loss or other unwanted behavior.
Confirming Navigation with CanDeactivate Guard
In Angular, guards are an essential part of the routing functionality, allowing developers to control access to certain routes in their applications. One common use case for guards is to confirm navigation before allowing the user to leave a particular route. This is where the CanDeactivate guard comes into play. The CanDeactivate guard is used to confirm navigation away from a route by prompting the user with a confirmation dialog.
When a user attempts to navigate away from a route that is protected by the CanDeactivate guard, Angular will trigger the guard’s canDeactivate method. This method will return a value indicating whether the navigation should be allowed to proceed or not. If the method returns true, the user will be allowed to navigate away from the route. If it returns false, the user will be prompted with a confirmation dialog asking if they are sure they want to leave the route.
To implement the CanDeactivate guard in your Angular application, you need to create a guard class that implements the CanDeactivate interface. This interface requires the implementation of a canDeactivate method that takes two parameters: the component being navigated away from and the current route state. Within the canDeactivate method, you can perform any necessary checks or logic to determine if the user should be allowed to navigate away from the route.
Once you have implemented the CanDeactivate guard class, you can then add it to the route configuration for the routes you want to protect. By adding the CanDeactivate guard to a route, you can ensure that the user is prompted with a confirmation dialog before navigating away from that route. This can be useful for preventing users from accidentally leaving a form with unsaved changes or navigating away from a critical page without confirmation. By leveraging the CanDeactivate guard, you can provide a smoother and more intuitive user experience in your Angular application.
Handling Unsaved Changes with CanDeactivate Guard
In Angular, guards are used to control access to certain routes in an application. They can be used to protect routes from unauthorized access, validate user input, and handle unsaved changes before navigating away from a page. One of the most common use cases for guards is handling unsaved changes with the CanDeactivate guard.
The CanDeactivate guard is used to prevent a user from navigating away from a page if there are unsaved changes on the page. This is important because users can accidentally lose their work if they navigate away from a page without saving their changes. By implementing the CanDeactivate guard, you can prompt the user to confirm whether they want to leave the page with unsaved changes.
To implement the CanDeactivate guard, you need to create a guard service that implements the CanDeactivate interface. This interface has a single method, canDeactivate, which takes two arguments: the component that is being deactivated and the current route state. In the canDeactivate method, you can check if there are any unsaved changes on the page and return a boolean value indicating whether the user can navigate away from the page.
Once you have implemented the CanDeactivate guard service, you need to add it to the routing configuration for the component you want to protect. You can do this by adding a canDeactivate property to the route definition in the routing module and specifying the guard service as the value. This will ensure that the guard is triggered when the user tries to navigate away from the page.
In conclusion, the CanDeactivate guard is a useful tool for handling unsaved changes in an Angular application. By implementing this guard, you can prevent users from accidentally losing their work and give them the opportunity to save their changes before navigating away from a page. If you are an Angular programmer looking to improve the user experience in your application, mastering the CanDeactivate guard is a key skill to have.
5: Implementing Resolve Guard
Setting up Resolve Guard
In this subchapter, we will discuss the process of setting up Resolve Guard in Angular routing. Resolve Guard is a type of guard that can be used to fetch data before a route is activated. This can be particularly useful when you need to ensure that certain data is available before a component is displayed to the user.
To set up a Resolve Guard, you first need to create a new service that implements the Resolve interface. This interface requires you to implement a resolve method that returns an Observable or Promise of the data that you want to fetch.
Next, you need to add this service to the providers array in your module file. This will make the service available for injection into your routing configuration.
Once you have created your Resolve Guard service, you can then use it in your routing configuration by adding a resolve property to the route object. This property should be an object where the keys are the names of the data properties you want to fetch, and the values are the services that will fetch that data.
For example, if you have a route that displays a user profile and you need to fetch the user data before the route is activated, you can create a UserService that implements the Resolve interface and add it to the resolve property of the route object.
By setting up Resolve Guards in this way, you can ensure that your components have access to the data they need before they are displayed to the user. This can help improve the user experience and prevent errors that can occur when data is not available when a component is rendered.
Pre-fetching Data with Resolve Guard
In Angular, guards are a powerful feature that allows developers to control access to different parts of an application based on certain conditions. One type of guard that is commonly used in Angular routing is the resolve guard. This guard is used to pre-fetch data before a route is activated, ensuring that the necessary data is available when the component associated with the route is rendered.
The resolve guard is especially useful when working with routes that require data from an API or external source. By using the resolve guard, developers can ensure that the data is fetched before the route is activated, preventing any potential issues with data not being available when the component is rendered. This can help improve the user experience by ensuring that the page loads quickly and smoothly, without any delays due to data fetching.
To implement a resolve guard, developers need to create a service that will fetch the necessary data. This service is then injected into the resolve guard, which can then use it to fetch the data before activating the route. By using the resolve guard in this way, developers can ensure that the data is fetched only when it is needed, reducing unnecessary API calls and improving overall performance.
One important thing to note when using the resolve guard is that it can be used in conjunction with other guards, such as the canActivate guard. This allows developers to control access to a route based on both whether the data is available and whether the user has the necessary permissions to access the route. By combining guards in this way, developers can create powerful and flexible access control mechanisms for their applications.
Overall, the resolve guard is a valuable tool for Angular programmers who need to pre-fetch data before activating a route. By using the resolve guard, developers can ensure that the necessary data is available when the route is activated, improving the user experience and performance of their applications.
Error Handling with Resolve Guard
In Angular routing, guards are used to protect routes by implementing logic before allowing a user to navigate to a certain route. One type of guard that is commonly used for error handling is the Resolve Guard. The Resolve Guard is used to fetch data before a route is activated, ensuring that the necessary data is available for the component to render correctly. In this subchapter, we will explore how to handle errors effectively using the Resolve Guard in Angular routing.
When using the Resolve Guard for error handling, it is important to handle errors gracefully to provide a better user experience. One way to do this is by catching errors in the resolver service and returning a new Observable with a default value or error message. This way, the resolver service can still return data to the component, even if an error occurs during data fetching.
Another approach to error handling with Resolve Guard is to redirect the user to an error page when an error occurs. This can be achieved by returning a new Observable with an error object that contains information about the error, such as an error code or message. The component can then check for this error object and redirect the user to the error page accordingly.
It is also important to log errors when using the Resolve Guard for error handling. By logging errors, developers can easily identify and troubleshoot issues that occur during data fetching. Logging errors can be done using the console.log() function or a logging service, such as Angular’s built-in logging service.
In conclusion, the Resolve Guard is a powerful tool for error handling in Angular routing. By handling errors gracefully, redirecting users to error pages, and logging errors, developers can ensure a smooth user experience when navigating through an Angular application. By mastering error handling with Resolve Guard, Angular programmers can build robust and reliable applications that provide a seamless user experience.
6: Implementing CanLoad Guard
Setting up CanLoad Guard
One of the most important concepts in Angular routing is the use of guards. Guards are used to determine whether a user can navigate to a certain route or not based on certain conditions. One type of guard is the CanLoad Guard, which is used to prevent a module from being loaded until certain conditions are met.
To set up a CanLoad Guard in your Angular application, you first need to create a guard class that implements the CanLoad interface. This interface has a single method called canLoad, which takes in two parameters: route and segments. The route parameter represents the route that the user is trying to access, while the segments parameter represents the segments of the URL.
Once you have created your guard class, you need to provide it in the providers array of your module. This tells Angular to use this guard whenever a CanLoad guard is needed. You can provide the guard at the module level or at the route level, depending on your specific requirements.
Next, you need to add the CanLoad Guard to the routes array in your routing module. You can do this by adding a canActivate property to the route object and passing in an array of guards that need to be run before the route can be loaded. In this case, you would pass in your CanLoad Guard.
Finally, you can add any additional logic to your CanLoad Guard’s canLoad method to determine whether the route should be loaded or not. This could involve checking if the user is authenticated, if certain data is available, or any other conditions that need to be met before the module can be loaded.
In conclusion, setting up a CanLoad Guard in your Angular application is an important step in controlling access to certain modules based on specific conditions. By following the steps outlined in this subchapter, you can ensure that your application remains secure and only allows access to authorized users.
Lazy Loading Modules with CanLoad Guard
In the world of Angular programming, guards play a crucial role in protecting routes and controlling access to certain parts of an application. One type of guard that is commonly used is the CanLoad guard, which allows lazy loading of modules based on certain conditions. In this subchapter, we will dive deep into how to utilize the CanLoad guard to lazy load modules in your Angular application.
Lazy loading modules with the CanLoad guard is a powerful feature that can greatly improve the performance and efficiency of your Angular application. By using the CanLoad guard, you can prevent unnecessary loading of modules that are not immediately required, thereby reducing the initial load time of your application. This can result in a smoother user experience and faster page loading times.
To implement lazy loading with the CanLoad guard, you first need to create a CanLoad guard service that implements the CanLoad interface. This service will contain the logic to determine whether a module can be loaded or not based on certain conditions, such as user authentication status or permissions. Once you have created the CanLoad guard service, you can then apply it to the desired routes in your Angular application using the canActivate property in the route configuration.
By using the CanLoad guard to lazy load modules in your Angular application, you can improve the overall performance and efficiency of your application. This can lead to a better user experience and faster page loading times, especially for larger applications with multiple modules. Additionally, lazy loading modules with the CanLoad guard can help you manage and optimize the loading of resources in your application, ultimately making your Angular application more scalable and maintainable. Mastering the CanLoad guard is an essential skill for any Angular programmer looking to take their routing guards to the next level.
Preventing Unauthorized Access with CanLoad Guard
In Angular, guards are used to prevent unauthorized access to certain routes within an application. One of the most commonly used guards is CanLoad Guard, which is specifically designed to prevent the loading of lazy-loaded modules if certain conditions are not met. This guard is essential for ensuring that only authenticated users are able to access certain parts of an application, such as admin panels or premium content.
To implement CanLoad Guard in your Angular application, you first need to create a guard service that implements the CanLoad interface. This interface requires you to define a canLoad method that returns a boolean or an Observable indicating whether or not the user is allowed to load the lazy-loaded module.
Next, you need to add the CanLoad Guard to the routes that you want to protect by adding a canActivate property to the route configuration object. This property should be set to an array containing the CanLoad Guard service that you created earlier.
When a user tries to navigate to a route that is protected by the CanLoad Guard, the guard service will be called and the canLoad method will be executed. If the method returns false or an Observable that emits false, the lazy-loaded module will not be loaded and the user will be redirected to a different route or shown an error message.
Overall, CanLoad Guard is a powerful tool for preventing unauthorized access to certain parts of an Angular application. By implementing this guard, you can ensure that only authenticated users are able to access sensitive information or premium features, helping to keep your application secure and user data protected.
7: Best Practices for Using Angular Routing Guards
Using Multiple Guards in Routes
In Angular routing, guards are used to protect routes by implementing logic before allowing a user to navigate to a certain route. There are various types of guards in Angular, such as CanActivate, CanActivateChild, CanDeactivate, and CanLoad. These guards can be used individually or in combination to provide different levels of protection for your routes.
When it comes to using multiple guards in routes, you have the flexibility to chain guards together to create complex logic for route protection. This can be particularly useful when you need to check for multiple conditions before allowing a user to access a route. By combining guards, you can create a layered approach to route protection, ensuring that only users who meet all the specified criteria can access a particular route.
To use multiple guards in routes, you simply need to add them to the canActivate or canLoad property of your route configuration in your routing module. Guards are executed in the order in which they are listed, so it’s important to consider the order in which you want your guards to be executed. This allows you to control the flow of logic and ensure that each guard is evaluated in the correct sequence.
One common scenario where you might want to use multiple guards is when you need to check for both authentication and authorization before allowing a user to access a route. In this case, you can create an authentication guard to check if the user is logged in and an authorization guard to check if the user has the necessary permissions to access the route. By chaining these guards together, you can ensure that only authenticated users with the correct permissions can access the route.
Overall, using multiple guards in routes gives you the flexibility to create complex route protection logic in your Angular application. By combining guards and chaining them together, you can create a layered approach to route protection that allows you to control the flow of logic and ensure that only users who meet all the specified criteria can access a particular route. This can help you enhance the security and usability of your application while providing a seamless user experience for your Angular programmers.
Error Handling in Guards
Error handling is an essential aspect of working with guards in Angular routing. Guards are used to protect routes in an Angular application by checking certain conditions before allowing access to a specific route. However, sometimes errors can occur during the execution of a guard, and it is important to handle these errors appropriately.
One common way to handle errors in guards is by using the catchError operator from RxJS. This operator allows you to catch errors thrown by an observable and handle them in a specific way. For example, you can display an error message to the user or redirect them to a different route if an error occurs in a guard.
Another approach to error handling in guards is to use the tap operator to log errors to the console for debugging purposes. This can be particularly useful when trying to troubleshoot issues with guards in an Angular application. By logging errors to the console, you can quickly identify what went wrong and take appropriate action to fix the problem.
In addition to using RxJS operators for error handling, you can also create custom error handling logic in guards by using try-catch blocks. This allows you to catch and handle errors in a more granular way, giving you greater control over how errors are managed in your application. By implementing custom error handling logic in guards, you can ensure that your application remains stable and resilient in the face of unexpected errors.
Overall, error handling in guards is an important consideration for Angular programmers working with routing in their applications. By using RxJS operators, logging errors to the console, and creating custom error handling logic, you can effectively manage errors that occur in guards and provide a better user experience for your application. Remember to always test your error handling logic thoroughly to ensure that it works as expected in all scenarios.
Testing Angular Routing Guards
In the world of Angular programming, understanding routing guards is crucial for creating secure and efficient applications. Routing guards serve as checkpoints that can be used to control access to certain routes in an Angular application. These guards can be used to protect routes that require authentication, authorization, or any other specific conditions to be met before allowing access.
One common type of routing guard is the CanActivate guard, which is used to prevent a user from navigating to a certain route if certain conditions are not met. This guard can be used to check if a user is authenticated before allowing access to a protected route, for example. By implementing the CanActivate guard, Angular programmers can ensure that only authorized users are able to access certain parts of their application.
Another type of routing guard is the CanActivateChild guard, which is used to protect child routes within a parent route. This guard can be used to apply certain conditions to child routes, such as requiring authentication or authorization before allowing access. By using the CanActivateChild guard, Angular programmers can create nested routes that are protected by specific conditions, ensuring that only authorized users can access certain parts of the application.
In addition to the CanActivate and CanActivateChild guards, Angular programmers can also make use of the CanDeactivate guard, which is used to prevent a user from leaving a route if certain conditions are not met. This guard can be used to prompt the user with a confirmation dialog before navigating away from a form, for example. By implementing the CanDeactivate guard, Angular programmers can create a smoother user experience by preventing accidental navigation away from certain routes.
Overall, understanding how to use routing guards in Angular is essential for creating secure and efficient applications. By implementing guards such as CanActivate, CanActivateChild, and CanDeactivate, Angular programmers can control access to routes, protect sensitive information, and create a more user-friendly experience for their applications. With a comprehensive understanding of routing guards, Angular programmers can take their applications to the next level and ensure the security and efficiency of their code.
8: Advanced Topics in Angular Routing Guards
Custom Guards
In Angular, guards play a crucial role in controlling access to different parts of an application. They are used to protect routes from being accessed by unauthorized users. Custom guards, in particular, allow developers to create their own logic for determining whether a user should be granted access to a specific route. This subchapter will explore the concept of custom guards in Angular routing and how they can be implemented in a project.
Custom guards are classes that implement the CanActivate interface in Angular. This interface requires the implementation of a canActivate method, which returns a boolean value indicating whether the user should be allowed to access the route. By creating custom guards, developers can define their own rules for access control, such as checking for user roles, permissions, or authentication status.
To create a custom guard in Angular, developers can generate a new service using the Angular CLI. This service should implement the CanActivate interface and define the logic for determining whether the user should be allowed to access the route. Once the guard is created, it can be added to the routing configuration by specifying it in the canActivate property of the route definition.
Custom guards can be used in combination with other built-in guards in Angular, such as canActivateChild and canLoad, to create complex access control logic. By chaining multiple guards together, developers can create a layered approach to access control, where different guards are applied to different parts of the application based on specific requirements.
Overall, custom guards provide developers with a powerful tool for implementing access control in Angular applications. By creating custom logic for determining access to routes, developers can ensure that only authorized users are able to access sensitive parts of the application. This subchapter will provide practical examples and best practices for implementing custom guards in Angular routing to help developers master this important aspect of Angular development.
Nested Routes and Guards
Nested Routes and Guards are essential concepts in Angular routing that every Angular programmer should be familiar with. In this subchapter, we will explore how nested routes and guards work together to enhance the security and functionality of your Angular applications.
Nested routes allow you to define child routes within a parent route, creating a hierarchical structure for your application. This can be useful for organizing and managing complex applications with multiple views and components. By nesting routes, you can create a more modular and maintainable codebase, as each route can have its own set of guards and parameters.
Guards in Angular routing are used to protect routes from unauthorized access or to perform certain actions before a route is activated. There are four types of guards in Angular: CanActivate, CanActivateChild, CanDeactivate, and CanLoad. These guards can be used to restrict access to certain routes based on user permissions, authentication status, or other conditions.
When using nested routes, guards can be applied at both the parent and child route levels. This allows you to control access to specific views or components within your application, ensuring that only authorized users can navigate to certain areas of your site. By combining nested routes and guards, you can create a secure and seamless user experience that meets the needs of your application.
In this subchapter, we will provide practical examples and code snippets to demonstrate how to implement nested routes and guards in your Angular applications. We will also discuss best practices for using guards in combination with nested routes, and provide tips for optimizing the performance and security of your application. By mastering nested routes and guards, you can take your Angular programming skills to the next level and build robust, secure, and user-friendly applications.
Dynamically Assigning Guards
In Angular, guards are a powerful feature that allow developers to control access to certain routes based on specified criteria. These guards can be used to protect routes from unauthorized users, ensure data is loaded before navigating to a route, or even prompt the user for confirmation before leaving a page. One of the key benefits of using guards is that they allow for dynamic assignment, meaning you can determine which guard to apply based on runtime conditions.
One common use case for dynamically assigning guards is implementing role-based access control in your Angular application. By using guards such as CanActivate or CanLoad, you can check the user’s role or permissions and only allow access to certain routes if the user meets the necessary criteria. This can help improve the security of your application by preventing unauthorized users from accessing sensitive information or functionality.
To dynamically assign guards in Angular, you can use a variety of techniques such as creating a custom guard factory or using dependency injection to provide guards based on runtime conditions. For example, you could create a guard factory that takes in a role as a parameter and returns the appropriate guard based on the user’s role. This allows for greater flexibility and reusability in your guard implementation.
Another way to dynamically assign guards in Angular is by using route data to specify which guards should be applied to a particular route. By adding a data property to your route configuration, you can specify an array of guards that should be applied when navigating to that route. This allows for a more declarative approach to guard assignment and makes it easier to manage complex guard logic.
Overall, dynamically assigning guards in Angular can help you create more flexible and secure applications by allowing you to control access to routes based on runtime conditions. By leveraging the power of guards, you can enhance the user experience, improve the security of your application, and ensure that data is loaded and validated before navigating to a new route. Mastering the use of guards in Angular routing is essential for any Angular programmer looking to build robust and secure applications.
9: Conclusion
Recap of Angular Routing Guards
As an Angular programmer, it’s important to understand the concept of Angular routing guards and how they can be used to enhance the security and functionality of your Angular applications. In this subchapter, we will provide a recap of Angular routing guards and how they can be effectively implemented in your projects.
Angular routing guards are used to control access to different parts of your application based on certain conditions. There are four types of routing guards in Angular: CanActivate, CanActivateChild, CanDeactivate, and CanLoad. CanActivate is used to determine if a route can be activated, CanActivateChild is used to determine if a child route can be activated, CanDeactivate is used to determine if a route can be deactivated, and CanLoad is used to determine if a lazy-loaded module can be loaded.
To implement routing guards in your Angular application, you need to create a service that implements the necessary guard interface (e.g., CanActivate). You then need to provide this service as a provider in your module’s providers array. Finally, you need to add the guard to the route configuration for the route you want to protect by adding a canActivate property to the route object.
One common use case for routing guards is to restrict access to certain routes based on the user’s authentication status. For example, you can use the CanActivate guard to prevent unauthenticated users from accessing certain routes in your application. This can help improve the security of your application by ensuring that only authorized users can access sensitive information or perform certain actions.
Another use case for routing guards is to prevent users from navigating away from a page without saving their changes. You can use the CanDeactivate guard to prompt users with a confirmation dialog before they leave a page with unsaved changes. This can help prevent accidental data loss and improve the user experience of your application.
In conclusion, Angular routing guards are a powerful tool that can help you control access to different parts of your application and improve the security and functionality of your Angular projects. By understanding how to implement and use routing guards effectively, you can enhance the user experience of your applications and ensure that they are secure and reliable.
Next Steps for Mastering Angular Routing Guards
Now that you have a solid understanding of what guards are in Angular routing and how they are used, it’s time to take your skills to the next level. In this subchapter, we will explore some advanced techniques and best practices for mastering Angular routing guards.
One of the key next steps for mastering Angular routing guards is to familiarize yourself with the different types of guards available in Angular. There are four main types of guards: CanActivate, CanActivateChild, CanDeactivate, and CanLoad. Each of these guards serves a specific purpose and can be used to control access to different parts of your application.
Another important aspect of mastering Angular routing guards is understanding how to handle authentication and authorization in your application. You can use guards to restrict access to certain routes based on the user’s authentication status or role. By implementing guards effectively, you can create a secure and user-friendly experience for your application users.
In addition to authentication and authorization, you can also use guards to perform other tasks such as data validation, error handling, and route redirection. By leveraging the full power of Angular routing guards, you can enhance the overall functionality and user experience of your application.
To further enhance your skills in working with Angular routing guards, consider exploring advanced topics such as combining multiple guards, creating custom guards, and handling asynchronous operations within guards. By mastering these advanced techniques, you can take your Angular programming skills to the next level and become a more proficient and versatile developer.
In conclusion, mastering Angular routing guards is essential for creating secure, efficient, and user-friendly applications. By understanding the different types of guards, implementing authentication and authorization, and exploring advanced techniques, you can take your skills to the next level and become a more proficient Angular programmer. So, don’t hesitate to dive deeper into the world of Angular routing guards and unlock the full potential of your applications.