Skip to content
What Should A Java Programmer Know?
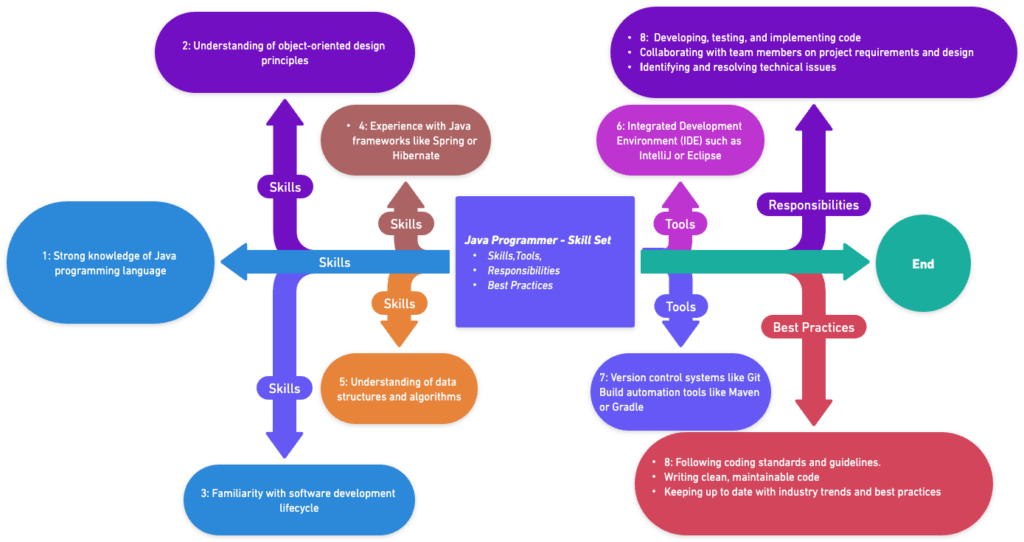
Basic Concepts & Language Fundamentals (1-20)
- What is Java and how does it work?
- Purpose: Assess understanding of Java basics and its operation (compilation, interpretation, JVM).
- Explain the concept of JVM, JRE, and JDK.
- Purpose: Test knowledge of Java’s runtime environment and development kit.
- What are the main features of Java?
- Purpose: Evaluate awareness of Java’s core features (e.g., object-oriented, platform-independent).
- Describe the data types in Java.
- Purpose: Assess understanding of Java’s primitive and non-primitive data types.
- Explain the use of
final
, finally
, and finalize
.
- Purpose: Distinguish between these keywords and their usage in Java.
- What is garbage collection in Java?
- Purpose: Evaluate understanding of memory management in Java.
- How do you manage memory in Java?
- Purpose: Test knowledge of memory management techniques, including garbage collection.
- Explain exception handling in Java.
- Purpose: Assess understanding of try-catch blocks, exception types.
- What are access modifiers in Java?
- Purpose: Evaluate knowledge of
public
, private
, protected
, and default access levels.
- Describe the Java Collections Framework.
- Purpose: Test understanding of collections and their hierarchy.
- What is the difference between
==
and .equals()
in Java?
- Purpose: Assess understanding of object equality and identity.
- Explain the concept of String Pool in Java.
- Purpose: Test knowledge of string internment and its benefits.
- How does Java implement polymorphism?
- Purpose: Evaluate understanding of static and dynamic polymorphism.
- What is multithreading in Java?
- Purpose: Assess knowledge of concurrent programming in Java.
- Describe synchronization in the context of multithreading.
- Purpose: Test understanding of thread safety and synchronization techniques.
- What is a Java annotation?
- Purpose: Evaluate understanding of annotations and their use cases.
- Explain the Java Memory Model.
- Purpose: Assess knowledge of how Java manages memory and concurrency.
- What are generics in Java?
- Purpose: Test understanding of type safety and generics.
- How do you handle null values in Java?
- Purpose: Evaluate strategies for dealing with null in Java applications.
- What is the difference between an abstract class and an interface?
- Purpose: Assess understanding of use cases for abstraction and interfaces.
Advanced Java Features & Best Practices (21-40)
- What are lambda expressions in Java?
- Purpose: Evaluate understanding of functional programming features in Java.
- Explain the Stream API in Java 8.
- Purpose: Test knowledge of stream operations and functional-style programming.
- What are the best practices for exception handling in Java?
- Purpose: Assess approaches to writing robust error handling code.
- How does Java handle concurrency and parallelism?
- Purpose: Evaluate understanding of concurrent and parallel programming models.
- What is the Java Native Interface (JNI)?
- Purpose: Test knowledge of integrating Java with other languages.
- Describe the role of the
volatile
keyword in Java.
- Purpose: Assess understanding of volatile variables in the context of concurrency.
- How do you ensure object immutability in Java?
- Purpose: Evaluate techniques for creating immutable objects.
- What is reflection in Java and how do you use it?
- Purpose: Test understanding of dynamic class manipulation and inspection.
- Explain dependency injection in Java.
- Purpose: Assess knowledge of dependency injection principles and frameworks.
- What is the role of the
transient
keyword in Java?
- Purpose: Evaluate understanding of object serialization.
- How do you implement RESTful services in Java?
- Purpose: Test knowledge of developing web services using Java technologies.
- What are design patterns and can you name a few common patterns in Java?
- Purpose: Assess understanding of design patterns and their applications.
- How do you manage transactions in Java?
- Purpose: Evaluate understanding of transaction management techniques.
- What are the new features introduced in Java 9/10/11/etc.?
- Purpose: Test knowledge of recent Java enhancements and their benefits.
- How do you optimize Java applications for performance?
- Purpose: Assess strategies for improving Java application performance.
- What is the role of the
@Deprecated
annotation?
- Purpose: Evaluate understanding of indicating deprecated APIs or features.
- Explain the differences between checked and unchecked exceptions.
- Purpose: Test understanding of exception categories in Java.
- What are the principles of Object-Oriented Programming (OOP)?
- Purpose: Assess knowledge of OOP fundamentals (encapsulation, inheritance, polymorphism, abstraction).
- How do you ensure code quality and maintainability in Java?
- Purpose: Evaluate practices for writing clean, maintainable code.
- What is the role of the
static
keyword in Java?
- Purpose: Test understanding of static context – methods, variables, blocks.
Java Frameworks & Libraries (41-60)
- What is Spring Framework and what are its core features?
- Purpose: Assess knowledge of the Spring Framework and its ecosystem.
- Explain the Spring Boot auto-configuration.
- Purpose: Test understanding of Spring Boot’s convention over configuration.
- How do you handle security in a Java application?
- Purpose: Evaluate strategies for securing Java applications (Spring Security, etc.).
- What is Hibernate and how does it relate to JPA?
- Purpose: Assess knowledge of ORM and database interaction in Java.
- Describe the concept of Aspect-Oriented Programming (AOP).
- Purpose: Test understanding of AOP and its implementation in Spring.
- How do you manage application profiles in Spring?
- Purpose: Evaluate techniques for configuring Spring applications in different environments.
- What is the purpose of the
@Transactional
annotation in Spring?
- Purpose: Assess understanding of declarative transaction management.
- Explain microservices architecture and its implementation with Spring Boot.
- Purpose: Test knowledge of developing microservices-based applications.
- What is Apache Kafka and how do you use it in Java applications?
- Purpose: Evaluate understanding of message brokers and event streaming.
- How do you monitor and manage Java applications in production?
- Purpose: Assess strategies for application monitoring and management (JMX, Spring Actuator).
Testing & Version Control (61-80)
- How do you write unit tests in Java?
- Purpose: Evaluate knowledge of unit testing frameworks (JUnit, TestNG).
- Explain integration testing in Java.
- Purpose: Test understanding of integration testing strategies and tools.
- What is Mockito and how do you use it?
- Purpose: Assess knowledge of mocking frameworks for testing.
- How do you manage version control for Java projects?
- Purpose: Evaluate use of version control systems (Git, SVN).
- What is Continuous Integration (CI) and how do you implement it in Java projects?
- Purpose: Test understanding of CI practices and tools (Jenkins, Travis CI).
- Explain the role of build tools in Java (Maven, Gradle).
- Purpose: Assess knowledge of dependency management and build automation.
- How do you debug Java applications?
- Purpose: Evaluate strategies for troubleshooting and debugging.
- What is code coverage and how do you measure it in Java?
- Purpose: Test understanding of code coverage metrics and tools.
- Describe the process of code review in your Java projects.
- Purpose: Assess practices for ensuring code quality through reviews.
- How do you document Java code and APIs?
- Purpose: Evaluate approaches to code documentation (Javadoc, Swagger).
Performance Tuning & Optimization (81-100)
- How do you identify and solve memory leaks in Java?
- Purpose: Assess strategies for detecting and resolving memory issues.
- Explain Java’s Just-In-Time (JIT) compiler.
- Purpose: Test knowledge of JIT compilation and its impact on performance.
- What are the best practices for using threads and concurrency in Java?
- Purpose: Evaluate understanding of concurrent programming best practices.
- How do you use profilers and other tools to optimize Java applications?
- Purpose: Assess knowledge of performance profiling and optimization tools.
- What is garbage collection tuning in Java?
- Purpose: Test understanding of GC algorithms and tuning techniques.
- How do you handle high-volume data processing in Java?
- Purpose: Evaluate strategies for processing large datasets efficiently.
- What is the NIO (New Input/Output) package and how does it improve performance?
- Purpose: Assess knowledge of non-blocking I/O operations.
- How do you optimize database interactions in Java applications?
- Purpose: Test understanding of optimizing database access and queries.
- What are JVM options you commonly adjust to optimize application performance?
- Purpose: Evaluate familiarity with JVM configuration for performance tuning.
- How do you manage and optimize application dependencies in Java?
- Purpose: Assess strategies for dependency management and reduction.